PHP: Removing Duplicated Array Values
How to remove duplicated array values in PHP and re-generate the array keys.

Edited: 2021-03-07 10:04
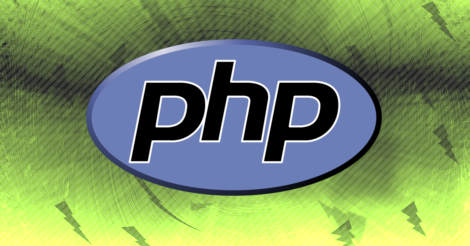
There are several ways to remove duplicated array values in PHP, and some might be less efficient than others, depending on the PHP version.
However, efficiency is only one thing to consider, and as mentioned again and again, it usually will not matter when you have a proper caching mechanism. Sometimes it does matter, so in general, you might as well just use the best method from the start, also since you might not be able to predict when or if someone will use your code somewhere where speed actually matters.
Another thing to consider is developer errors due to poor implementation. Some methods to remove duplicated array values could lead to missing numeric keys, which might cause bugs down the road. It is a best practice to code around such problems whenever possible.
Now, if you just need to get rid of duplicated array values, and you do not care about preserving the array keys, you should probably be using a combination of array_key and array_flip, since some benchmarks have shown this to be the fastest method.
$some_array = array('array_keys', 'array_keys', 'array_flip', 'array_unique', 'array_search', 'array_values', 'array_unique');
$unique_array = array_keys(array_flip($some_array));
print_r($unique_array);
Output:
Array
(
[0] => array_keys
[1] => array_flip
[2] => array_unique
[3] => array_search
[4] => array_values
)
Remove duplicates while preserving keys
To preserve the array keys, you can use the double flip method:
$some_array = array('Apple', 'Apple', 'Banana', 'Orange', 'Carrot', 'Carrot', 'Spinach');
$unique_array = array_flip(array_flip($some_array));
print_r($unique_array);
However, to iterate over an array that has missing keys, you should use foreach rather than while and for loops:
foreach ($some_array as $key => $value) {
echo PHP_EOL . $key . ':' . $value;
}
Output
0:Apple
1:Banana
2:Orange
3:Carrot
4:Spinach
This works for both numeric arrays and associative arrays.
Tell us what you think: