Get a Random Value From an Array in PHP
Tutorial on how to obtain a random value from an array in PHP.

Edited: 2021-03-07 10:03
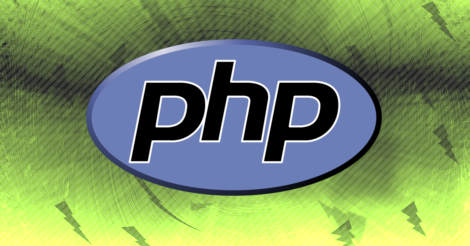
In this tutorial, you will learn how to get a random value from an array in PHP.
There actually is a build-in function we can use for this, array_rand, which works for both numeric and and string keys. This is probably the preferred way in most circumstances, since it works for both indexed- and associative arrays.
We can leverage array_rand like this:
$cars_array = array('some string key' => 'Acura', 'BMW', 'Tesla', 'Audi');
$key = array_rand($cars_array);
echo $arr["$key"];
Random array value with Shuffle and Rand
If you want to get really fancy, you could also use the shuffle function to randomize the order of all the elements in an array (not recommended):
$cars_array = array('Buick', 'Bentley', 'just a string key' => 'Chevrolet', 'Cadillac');
shuffle($cars_array);
$first_key = array_key_first($cars_array);
echo $arr["$first_key"];
Because the array is shuffled, and depending on the size of the array, this method is going to be slower. But, we should get a new value most of the time. The use of array_key_first makes the code work for both integer keys and string keys, which is good when aiming to maximize the portability of your code.
Note. Shuffle breaks the association of kays and values, assigning new keys to each value. You may therefor want to avoid using this function, as it has poor portability.
The final method, making use of rand, is limited to numeric array keys. This works since we are generating a random number between 0 and the size of the array. We can know the size of the array by using the count function to return the number of elements in the array:
$cars_array = array('Ford', 'Toyota', 'Volkswagen', 'Mazda');
$random_number = rand(0, count($cars_array));
echo $cars_array["$random_number"];
Tell us what you think: