Foreach With Square Bracket Syntax in PHP
Tutorial on how to traverse a Multidimensional array using php7.1 syntax

Edited: 2021-03-07 10:03
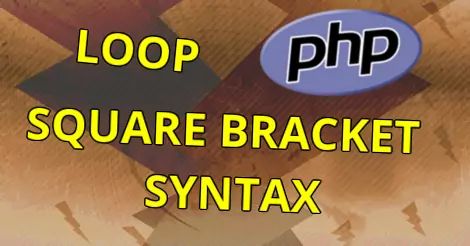
PHP 7.1 introduced a useful new syntax to destructure an array into variables, and iterate over multidimensional arrays.
It involves the clever use of square brackets [ and ] inside a single foreach expression.
If the level of nesting is known, we can do with a single foreach loop—there is no longer a need to use nested loop structures to iterate over a multidimensional array. If we do not know the level of nesting before hand, we can still resort to old tactics. such as using a recursive function to loop over the array.
The syntax is best demonstrated in the below example:
foreach ($some_array as [
'name' => $name,
'email' => $email,
]) {
// foreach expressions:
echo $name . ':'. $email . PHP_EOL;
}
Brackets syntax for array destructuring
There is a few fully functioning examples included here in case you need it.
The example below uses the new bracket syntax on a 2-dimensional array:
$some_array[] = [
'name' => 'JacobSeated',
'email' => 'seat@example.com'
];
$some_array[] = [
'name' => 'Rasmus',
'email' => 'rasmus@example.com'
];
foreach ($some_array as [
'name' => $name,
'email' => $email,
]) {
// foreach expressions:
echo $name . ':'. $email . PHP_EOL;
}
Finally, if you have a 3-level array you would simply use the same syntax, as when assigning values to an array. The below may be used on a 3-dimensional array:
$some_array[] = [
'name' => 'Sophia',
'email' => 'sophia@example.com',
'more' => [
'title' => 'I like cake',
'content' => 'Lots of sugar'
]
];
$some_array[] = [
'name' => 'Andrea',
'email' => 'andrea@example.com',
'more' => [
'title' => 'anything good and happy',
'content' => 'I am content with using PHP'
]
];
foreach ($some_array as [
'name' => $name,
'email' => $email,
'more' => [
'content' => $content,
'title' => $title
]])
{
// Foreach expressions:
echo $name . ':'. $content . PHP_EOL;
}
Tell us what you think: