Reordering Array Elements in PHP
How to reorder array elements in PHP, moving elements up or down one step at a time.

Edited: 2021-03-07 10:03
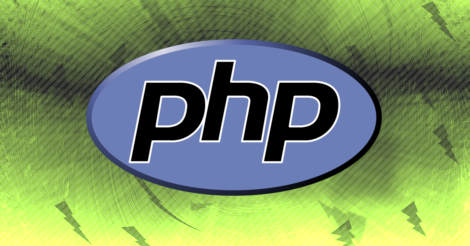
Moving a single array element up or down one step at a time is actually not that easy. I wonder why there is no build-in function for this in PHP, as it is a very common thing to want to do.
I came up with a function for reordering arrays that can both move elements up and down. It works for both associative- and indexed arrays, with strings, integers, and boolean values – and it preserves the keys as well.
This function can be used for reordering of photos and links on a website.
The easiest way to remember the ordered array is to convert the array to JSON and store it on the file system or in a database.
To use the function, simply do like this:
$contact_array = array(
'admin' => 'admin@example.com',
'webmaster' => 'webmaster@example.com',
'support' => 'support@example.com',
'reception' => 'reception@example.com',
);
// last parameter can either be "up" or "down"
$reordered = array_shove($contact_array, 'webmaster', 'down');
// Show the re-ordered array
print_r($reordered);
If you want to store the array as JSON:
echo json_encode($reordered);
Note. It is recommended to use a file handler class in concurrency situations.
The function itself:
function array_shove(array $array, $selected_key, $direction)
{
$new_array = array();
foreach ($array as $key => $value) {
if ($key !== $selected_key) {
$new_array["$key"] = $value;
$last = array('key' => $key, 'value' => $value);
unset($array["$key"]);
} else {
if ($direction !== 'up') {
// Value of next, moves pointer
$next_value = next($array);
// Key of next
$next_key = key($array);
// Check if $next_key is null,
// indicating there is no more elements in the array
if ($next_key !== null) {
// Add -next- to $new_array, keeping -current- in $array
$new_array["$next_key"] = $next_value;
unset($array["$next_key"]);
}
} else {
if (isset($last['key'])) {
unset($new_array["{$last['key']}"]);
}
// Add current $array element to $new_array
$new_array["$key"] = $value;
// Re-add $last to $new_array
$new_array["{$last['key']}"] = $last['value'];
}
// Merge new and old array
return $new_array + $array;
}
}
}
Reordering arrays
To reorder the array, I came up with a function that iterates over (loops through) the array.
While the loop is running, I check if the $selected_key has been reached on each run. When the $selected_key is reached, I add the $new_array with the $array and return the result.
$new_array is a temporary variable containing the elements we have gone through so far, while the $array variable contains what is left.
The rest of the code deals with taking the $selected_key element and adding it either before the last element if we are going up or after the next element if we are going down.
Tell us what you think: