Random Number Generator in PHP
How to generate and use random numbers for different purposes in PHP.
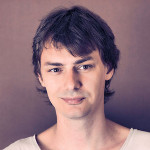
By. Jacob
Edited: 2021-02-14 05:34
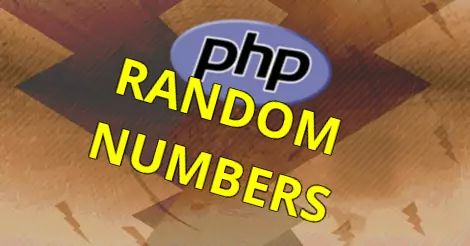
PHP comes with its own function for generating random numbers, so there is really not any reason to create your own algorithm.
Of course, when we talk about "random numbers", you should remember that there really is not such thing as a truly random number; in this context, "random" just means that we can not predict what the number is going to be, since we do not know the state of the algorithm used to generate the number. It is, of course, an irrelevant technical detail that most of us never have to think about.
Random numbers can be generated many different ways, some of which use external input as a "seed" value for the number. If you were to create a random number generator yourself, then you might use the current date, hour, or second as a seed value.
Now, it happens that PHP already has a function for generating random numbers, rand, which you can use, so there is no reason to create your own. Here is an example:
echo rand(1, 20); // Outputs a whole number E.g.: 1, 5, 20
Showing a random message
One thing that generating random numbers is useful for, is when you want to display a random message to the user; an easy way to do this is by having an indexed array containing the items you want to randomly choose from.
$items = [];
$items[] = 'Welcome to my website young one.';
$items[] = 'Today is a good day.';
$items[] = 'Fantastic entrance. Welcome. Have a look around.';
$items[] = 'Welcome. No funny business!';
$i = count($items);
$picked_item = rand(0, $i-1);
echo $items["$picked_item"];
Since an indexed array begins from "0", you will have to subtract "1" from the, $i, count variable.
It should be relatively easy for you to change this to instead show a random image; or, if you feel like it, you can also just read this tutorial: Show random image with PHP
Links
- rand - php.net
Tell us what you think: