Detect the request method in PHP
Detecting the request method used to fetch a web page with PHP, routing, HTTP responses, and more.
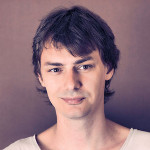
By. Jacob
Edited: 2021-04-22 09:52
PHP makes it easy to detect the request method via the $_SERVER['REQUEST_METHOD'] superglobal, which is useful for URL mapping (routing) purposes; to do so, you just need to compare values using a if statement, so, to check if the request is a POST:
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
echo 'Dealing with a POST request.';
exit();
}
And, if you want to check if the request is a GET request:
if ($_SERVER['REQUEST_METHOD'] === 'GET') {
echo 'Dealing with a GET request.';
exit();
}
Disallowing certain request types
You can accept or deny the request depending on whether the used request method is allowed for the requested path.
If you deny a request, then it is important to send an appropriate response code in order to adhere to the HTTP protocol; for example, an accurate status code to send if a used request method is not allowed would the 405 Method Not Allowed. E.g:
http_response_code(405);
header('Allow: GET, HEAD');
echo '<h1>405 Method Not Allowed</h1>';
exit();
You can keep a list of allowed methods in an Associative Array that you can check the $_SERVER['REQUEST_METHOD'] against. E.g:
$allowed_methods = ['GET', 'HEAD'];
if (!in_array($_SERVER['REQUEST_METHOD'], $allowed_methods)) {
http_response_code(405);
header('Allow: '. implode(", ", $allowed_methods));
echo '<h1>405 Method Not Allowed</h1>';
exit();
}
The same technique, with Associative Arrays, can also be used to compare the requested path with a list of mapped URLs, but you probably also want to match patterns, which is slightly more complex.
HTTP request types:
- GET
- POST
- HEAD
- PUT
- DELETE
- PATCH
Routing
If you are trying to create a URL mapper (router), then it will often make more sense to make a single script or class that handles all HTTP requests for your application; in order for that to work, you will first need to point all requests to the relevant script (E.g. index.php), and then parse the path from within your application.
The path is contained in the $_SERVER['REQUEST_URI'] variable, which also contains the query string, and therefor it needs to be parsed before you can use it. E.g:
$parsed_url = parse_url($_SERVER['REQUEST_URI']);
if ($parsed_url['path'] === '/my/unique-path') {
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
echo 'Dealing with a POST request.';
exit();
}
}
Note. the query string is the part after the question mark in the URL. E.g: /some-user?user_name=Henrik&category=employees.
Ideally, this should be created in a much more dynamic way, so that the script automatically loads the feature that corresponds with the path and/or used URL parameters included in the request.
More info: How to create a router in PHP
Handle all requests from a single PHP script
It is possible to direct all HTTP requests to go through a single PHP script, how to do this will depend on the web server software you are using; if you are using the Apache HTTP server, then you just need to add the following to your VHOST or .htaccess configuration:
RewriteEngine on
RewriteCond %{REQUEST_FILENAME} !-f
RewriteCond %{REQUEST_FILENAME} !-d
RewriteRule ^.*$ index.php [QSA,L]
This will make all requests go through your index.php file unless a physical file exists in the sites web folder; if you also want to serve physical files through PHP, then you will need to use a slightly different configuration:
RewriteEngine on
RewriteCond %{REQUEST_FILENAME} -f [OR]
RewriteCond %{REQUEST_FILENAME} !-f
RewriteRule ^.*$ index.php [QSA,L]
More info: Redirect Everything to Index.php With Mod_rewrite and How to create a router in PHP
Note. You can use Beamtic's file handler class to serve static files through PHP, more info is found here.
Tell us what you think: